Service Definition
With your service opened in its Devcontainer, you are ready to start developing. The first step is to define how your service can be configured and how it interacts with other services.
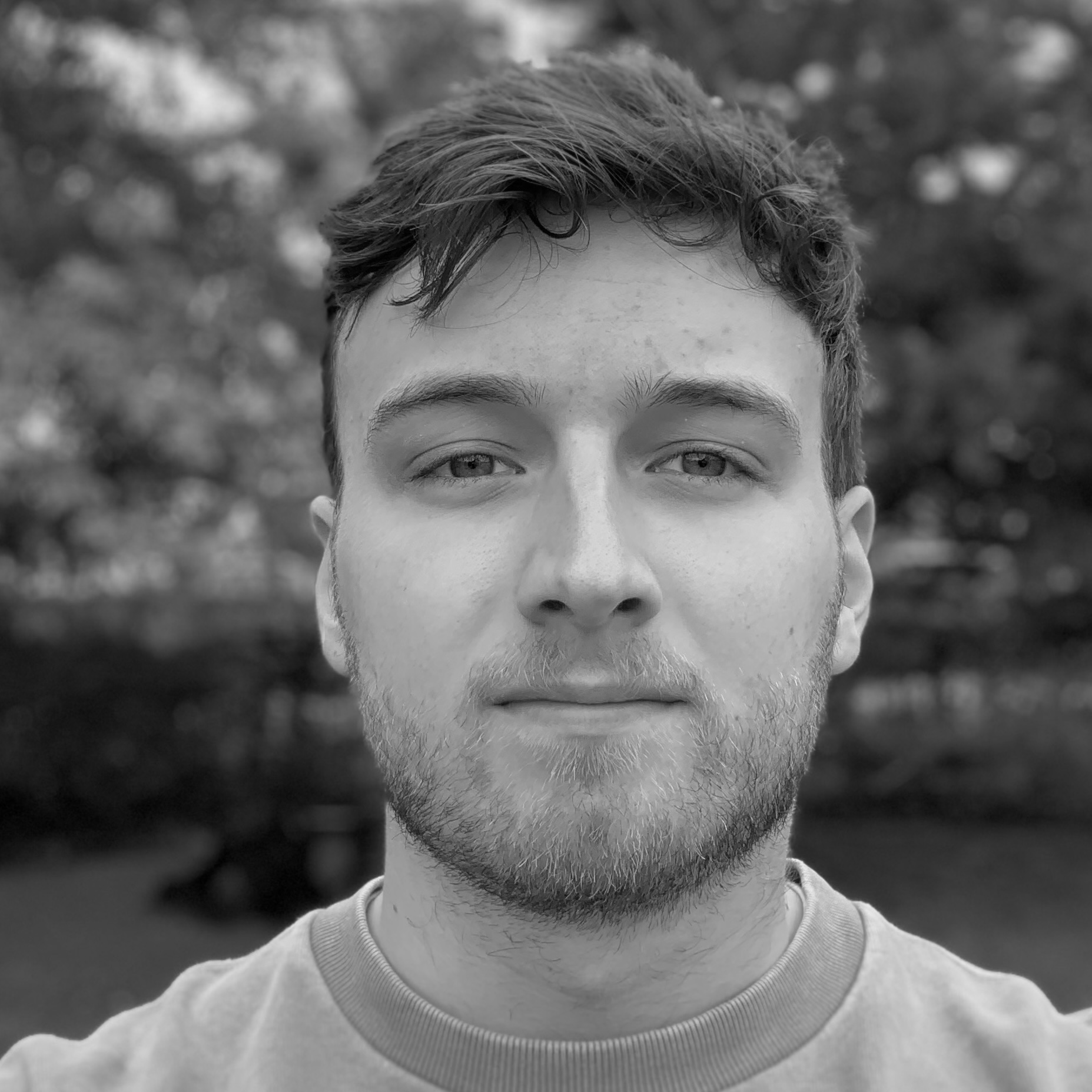
Elias Groot
Software Lead, Project Administrator
Prerequisites
- You have
roverctl
and Docker installed - You have VS Code and the Devcontainer plugin installed
- You cloned a service template using
roverctl
and opened it in its Devcontainer
How Services Are Built and Run
Each service is built and run by roverd
, a process that is always running on the Rover. roverd
is responsible for managing service files, validating pipelines and keeping track of service execution. It is the most vital piece of software in the ASE framework, even though you will most likely never interact with it manually.
roverd
exposes an HTTP REST API. This means that you can start services, view pipelines and query logs with GET
and POST
requests to your Rover. This is how roverctl
and roverctl-web
work.
The set up and terminology might ring a bell for those familiar with Kubernetes, from which we took inspiration.
Using service.yaml Files
In order for roverd
to understand how a service communicates with other services and how it should be executed and run, we use service.yaml files. You will see them a lot.
Each service should have exactly one service.yaml file at the root of its service folder:
/my-first-service
├── service.yaml
├── /src
├── source.code
├── docs.md
...
Service Identity
Locate and open the service.yaml that comes with the template that you just initialized. Notice the author
, name
and version
fields. Combined, they make up for a service's fully qualified name, or FQN in short. This FQN is unique and determines how the service is stored on the Rover.
# Service identity
name: my-example service
author: elias
source: https://github.com/elias/my-example-service # use this for bookkeeping, to tie service files to git repositories
version: 1.0.0
...
Keep in mind that a good service never runs alone: services are meant to be chained together in a pipeline managed by roverd
. Communication between services is name-bound, so no two services with the same effective name can be enabled at the same time.
Service Commands
Because services are Linux processes that can be created in any language, there is no standard way to build or run a service. We need to help roverd
by specifying the commands to use in the commands
block in our service.yaml.
- Go
- Python
- C
- C++
- Other languages
...
commands:
build: make build
run: ./bin/my-example-service
...
Commands are run by roverd
using a login shell for the standard user "debix", executed in the service directory. The above commands would be executed as follows by roverd
:
Build
# Executed on the Rover by roverd
cd /home/debix/.rover/author/name/version # path is based on the service FQN
make build
Run
# Executed on the Rover by roverd
cd /home/debix/.rover/author/name/version # path is based on the service FQN
./bin/my-example-service
...
commands:
build: echo "no build command needed"
run: ./src/main.py
...
Commands are run by roverd
using a login shell for the standard user "debix", executed in the service directory. The above commands would be executed as follows by roverd
:
Build
# Executed on the Rover by roverd
cd /home/debix/.rover/author/name/version # path is based on the service FQN
echo "no build command needed"
Run
# Executed on the Rover by roverd
cd /home/debix/.rover/author/name/version # path is based on the service FQN
./src/main.py
...
commands:
build: make build
run: ./bin/my-example-service
...
Commands are run by roverd
using a login shell for the standard user "debix", executed in the service directory. The above commands would be executed as follows by roverd
:
Build
# Executed on the Rover by roverd
cd /home/debix/.rover/author/name/version # path is based on the service FQN
make build
Run
# Executed on the Rover by roverd
cd /home/debix/.rover/author/name/version # path is based on the service FQN
./bin/my-example-service
...
commands:
build: make build
run: ./bin/my-example-service
...
Commands are run by roverd
using a login shell for the standard user "debix", executed in the service directory. The above commands would be executed as follows by roverd
:
Build
# Executed on the Rover by roverd
cd /home/debix/.rover/author/name/version # path is based on the service FQN
make build
Run
# Executed on the Rover by roverd
cd /home/debix/.rover/author/name/version # path is based on the service FQN
./bin/my-example-service
...
commands:
build: compile.sh # this can be any valid bash command
run: run.sh # this can be any valid bash command
...
Commands are run by roverd
using a login shell for the standard user "debix", executed in the service directory. The above commands would be executed as follows by roverd
:
Build
# Executed on the Rover by roverd
cd /home/debix/.rover/author/name/version # path is based on the service FQN
./compile.sh
Run
# Executed on the Rover by roverd
cd /home/debix/.rover/author/name/version # path is based on the service FQN
./run.sh
All build
and run
commands should be valid bash commands. You can read more about the buildtime and run-time environment here.
Accessing Service Information in Code
The service.yaml file is a static definition that can be accessed in code using the APIs that the roverlib provides for your language. How this is done, depends on the chosen language.
- Go
- Python
- C
- C++
- Other languages
Open the main program in src/main.go.
roverlib-go
passes all service information to a MainCallback
function. You can then read and print the service identity like so:
func run(service roverlib.Service, configuration *roverlib.ServiceConfiguration) error {
log.Info().Msgf("Hello world, a new Go service '%s' was born at version %s", *service.Name, *service.Version)
}
Open the main program in src/main.py.
roverlib-python
passes all service information to a MainCallback
callable function. You can then read and print the service identity like so:
def run(service : roverlib.Service, configuration : roverlib.ServiceConfiguration):
logger.info(f"Hello World, a new Python service {service.name} was born at version {service.version}")
Open the main program in src/main.c.
roverlib-c
passes all service information to a *Main_callback
function pointer. You can then read and print the service identity like so:
int user_program(Service service, Service_configuration *configuration) {
printf("Hello world, a new C service '%s' was born at version %s\n", service.name, service.version);
}
Open the main program in src/main.cpp.
The C++ template usesroverlib-c
, which passes all service information to a *Main_callback
function pointer. You can then read and print the service identity like so:
int user_program(Service service, Service_configuration *configuration) {
printf("Hello world, a new C service '%s' was born at version %s\n", service.name, service.version);
}
You will need to parse the service information that is injected through the ASE_SERVICE
environment variable manually. This environment variable contains the bootspec which you need to represent in the native format of your language.
There are many more APIs that the roverlib provides, which we will go over step by step.