Write to a Service
Similar to reading, services can output data on write streams for other services to read. We will write "decision" outputs to steer the Rover.
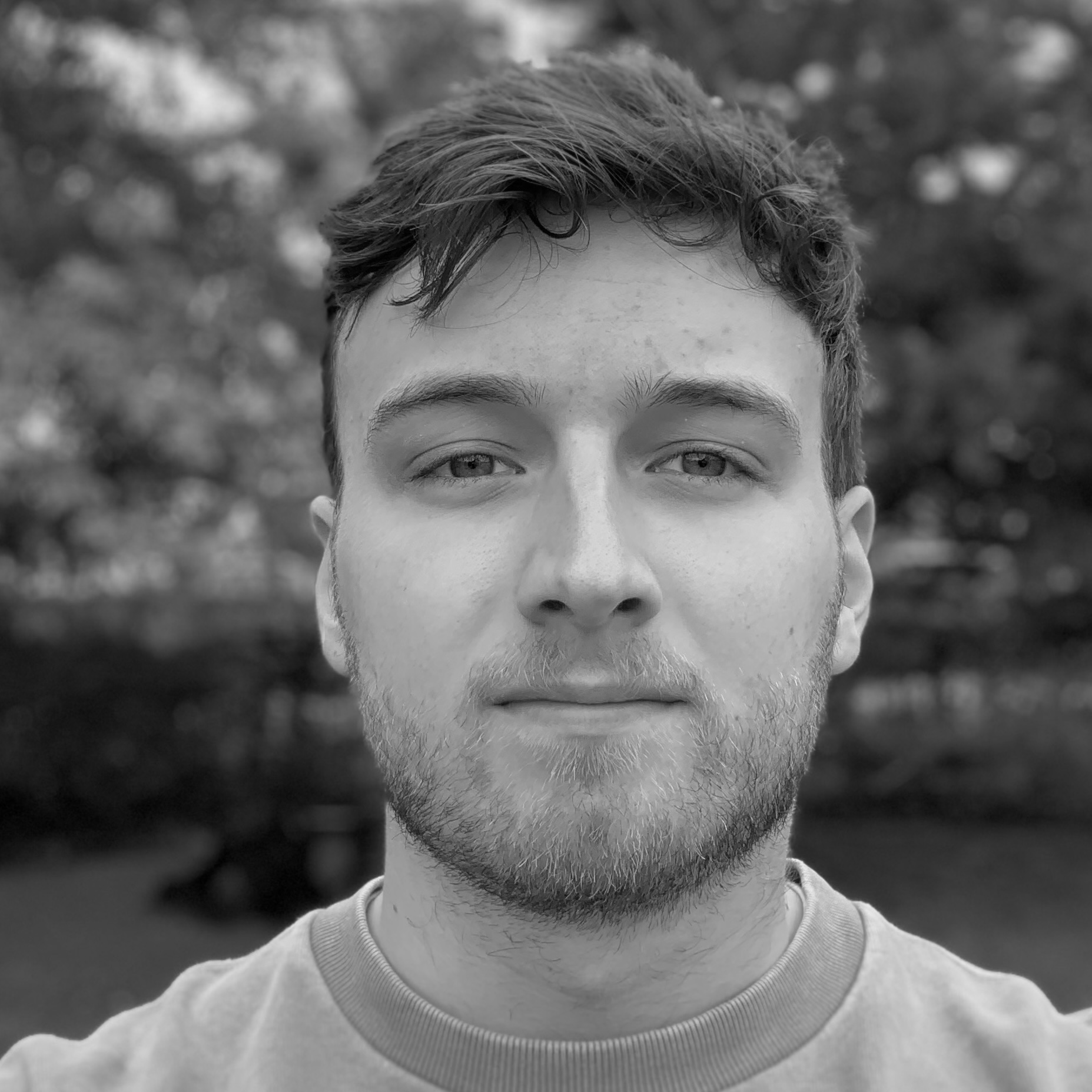
Elias Groot
Software Lead, Project Administrator
Prerequisites
- You have
roverctl
and Docker installed - You have VS Code and the Devcontainer plugin installed
- You cloned a service template using
roverctl
and opened it in its Devcontainer - You are familiar with using
roverctl-web
to configure and execute a pipeline - You know how to upload your service using
roverctl
How to Write
Any service can output data to 0 or more output/write streams. Just like with input/read streams, these must be declared in the service.yaml file. Open your template service's declaration.
...
outputs:
- decision
...
The above tells us that our service outputs data to the "decision" stream. All outputs stream follow a one-to-many principle: 0 or more other services could "subscribe" to our output stream and read from it.
To write data to this stream in your service's source code, you can open a write stream and write using the APIs provided by roverlib for your language. Again, it is recommended to communicate using our standard messaging definitions but you can also send raw bytes if necessary.
- Go
- Python
- C
- C++
- Other languages
roverlib-go
exposes the GetWriteStream()
method, that can be used to open a write stream that corresponds to the one defined in the service.yaml file, like so:
func run(service roverlib.Service, configuration *roverlib.ServiceConfiguration) error {
writeStream := service.GetWriteStream("decision")
if writeStream == nil {
return fmt.Errorf("Failed to create write stream 'decision'")
}
actuatorMsg := pb_outputs.SensorOutput{
Timestamp: uint64(time.Now().UnixMilli()), // milliseconds since epoch
Status: 0, // all is well
SensorId: 1,
SensorOutput: &pb_outputs.SensorOutput_ControllerOutput{
ControllerOutput: &pb_outputs.ControllerOutput{
SteeringAngle: steerPosition,
LeftThrottle: float32(tunableSpeed),
RightThrottle: float32(tunableSpeed),
FanSpeed: 0,
FrontLights: false,
},
},
}
err = writeStream.Write(&actuatorMsg)
if err != nil {
log.Warn().Err(err).Msg("Could not write")
}
}
Or, to write bytes:
func run(service roverlib.Service, configuration *roverlib.ServiceConfiguration) error {
writeStream := service.GetWriteStream("decision")
if writeStream == nil {
return fmt.Errorf("Failed to create write stream 'decision'")
}
err = writeStream.WriteBytes([]byte{"hello, world"})
if err != nil {
log.Warn().Err(err).Msg("Could not write")
}
}
roverlib-python
exposes the GetWriteStream()
method, that can be used to open a write stream that corresponds to the one defined in the service.yaml file, like so:
def run(service : roverlib.Service, configuration : roverlib.ServiceConfiguration):
write_stream : roverlib.WriteStream = service.GetWriteStream("decision")
if write_stream is None:
raise ValueError("Failed to create write stream 'decision'")
write_stream.Write(rovercom.SensorOutput(
sensor_id=1,
timestamp=int(time.time() * 1000),
controller_output=rovercom.ControllerOutput(
steering_angle = steer_position,
left_throttle = tunable_speed,
right_throttle = tunable_speed,
)
))
Or, to write bytes:
def run(service : roverlib.Service, configuration : roverlib.ServiceConfiguration):
write_stream : roverlib.WriteStream = service.GetWriteStream("decision")
if write_stream is None:
raise ValueError("Failed to create write stream 'decision'")
write_stream.WriteBytes(b"hello, world")
roverlib-c
exposes the get_write_stream()
function, that can be used to open a write stream that corresponds to the one defined in the service.yaml file, like so:
int user_program(Service service, Service_configuration *configuration) {
write_stream *write_stream = get_write_stream(&service, "decision");
if (write_stream == NULL) {
printf("Failed to create write stream 'decision'\n");
}
// Initialize the message that we want to send to the actuator
ProtobufMsgs__SensorOutput actuator_msg = PROTOBUF_MSGS__SENSOR_OUTPUT__INIT;
// Set the message fields
actuator_msg.timestamp = current_time_millis(); // milliseconds since epoch
actuator_msg.status = 0; // all is well
actuator_msg.sensorid = 1;
// Set the oneof field contents
ProtobufMsgs__ControllerOutput controller_output = PROTOBUF_MSGS__CONTROLLER_OUTPUT__INIT;
controller_output.steeringangle = steer_position;
controller_output.leftthrottle = *tunable_speed;
controller_output.rightthrottle = *tunable_speed;
controller_output.fanspeed = 0;
controller_output.frontlights = false;
// Set the oneof field (union)
actuator_msg.controlleroutput = &controller_output;
actuator_msg.sensor_output_case = PROTOBUF_MSGS__SENSOR_OUTPUT__SENSOR_OUTPUT_CONTROLLER_OUTPUT;
int res = write_pb(write_stream, &actuator_msg);
if (res <= 0) {
printf("Could not write\n");
return 1;
}
}
Or, to write bytes:
int user_program(Service service, Service_configuration *configuration) {
write_stream *write_stream = get_write_stream(&service, "decision");
if (write_stream == NULL) {
printf("Failed to create write stream 'decision'\n");
}
char buf[4096];
int res = write_bytes(write_stream, buf);
if (res <= 0) {
printf("Could not write\n");
return 1;
}
}
The C++ template uses roverlib-c
, which exposes the get_write_stream()
function, that can be used to open a write stream that corresponds to the one defined in the service.yaml file, like so:
int user_program(Service service, Service_configuration *configuration) {
write_stream *write_stream = get_write_stream(&service, "decision");
if (write_stream == NULL) {
printf("Failed to create write stream 'decision'\n");
}
// Initialize the message that we want to send to the actuator
ProtobufMsgs__SensorOutput actuator_msg = PROTOBUF_MSGS__SENSOR_OUTPUT__INIT;
// Set the message fields
actuator_msg.timestamp = current_time_millis(); // milliseconds since epoch
actuator_msg.status = 0; // all is well
actuator_msg.sensorid = 1;
// Set the oneof field contents
ProtobufMsgs__ControllerOutput controller_output = PROTOBUF_MSGS__CONTROLLER_OUTPUT__INIT;
controller_output.steeringangle = steer_position;
controller_output.leftthrottle = *tunable_speed;
controller_output.rightthrottle = *tunable_speed;
controller_output.fanspeed = 0;
controller_output.frontlights = false;
// Set the oneof field (union)
actuator_msg.controlleroutput = &controller_output;
actuator_msg.sensor_output_case = PROTOBUF_MSGS__SENSOR_OUTPUT__SENSOR_OUTPUT_CONTROLLER_OUTPUT;
int res = write_pb(write_stream, &actuator_msg);
if (res <= 0) {
printf("Could not write\n");
return 1;
}
}
Or, to write bytes:
int user_program(Service service, Service_configuration *configuration) {
write_stream *write_stream = get_write_stream(&service, "decision");
if (write_stream == NULL) {
printf("Failed to create write stream 'decision'\n");
}
char buf[4096];
int res = write_bytes(write_stream, buf);
if (res <= 0) {
printf("Could not write\n");
return 1;
}
}
You will need to parse the service information that is injected through the ASE_SERVICE
environment variable manually. This environment variable contains the bootspec which you need to represent in the native format of your language. Then, you need to open a ZMQ socket based on the correct stream properties.
Alter Steering
In your service template, try to alter the steering and throttle values that you write. Then, open your service.yaml file and bump the version number to 0.0.2.
Now, reupload your service and open roverctl-web
again. Notice that you can choose between multiple versions of the same service now. We recommend versioning your services wisely, so that you can quickly A/B-test and revert to old code.
Finally, start the pipeline and see how the changes in your source code impact how the Rover drives.
Debugging
Just like with the official ASE services, you can debug your output data if you use our communication definitions. Open roverctl-web
in debug mode and enable debug mode for your pipeline.
Start the pipeline again and notice that the output data by your template service is plotted there. If you do not use our communication definitions, you will lose this debugging functionality.