Tune to a Service
At this point, there is one remaining section in a service.yaml that needs discussing. The configuration
field. It allows for convenient configuration and over-the-air tuning of a service.
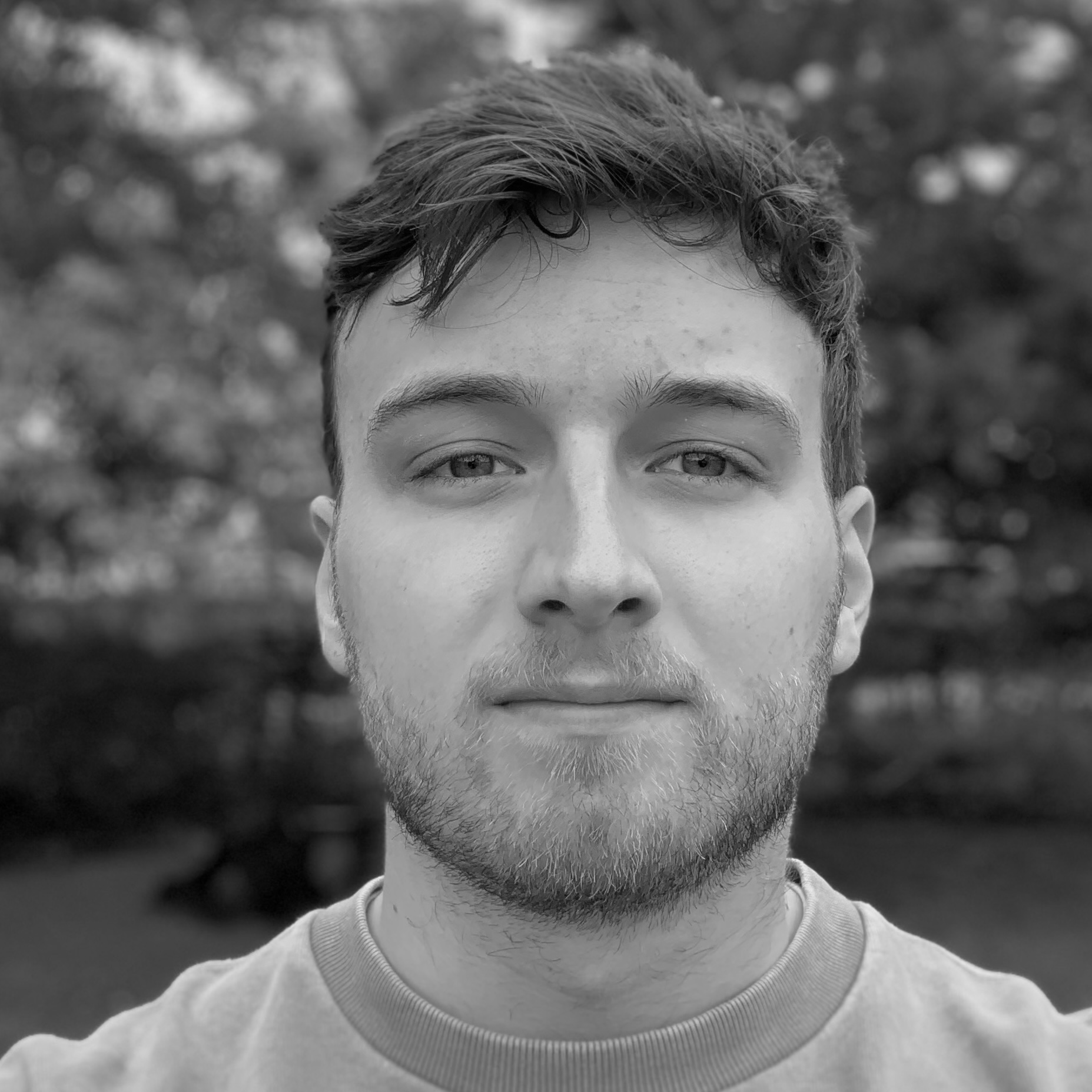
Elias Groot
Software Lead, Project Administrator
Prerequisites
- You have
roverctl
and Docker installed - You have VS Code and the Devcontainer plugin installed
- You cloned a service template using
roverctl
and opened it in its Devcontainer - You are familiar with using
roverctl-web
to configure and execute a pipeline - You know how to upload your service using
roverctl
Configuration Values
Instead of hardcoding configuration values in your service's source code, you can define them in your service.yaml. Then, you do not need to rebuild your service when altering configuration values. Open your template service's declaration.
...
configuration:
- name: speed
type: number
value: 0.2
tunable: true
The above tells us that there is one configuration value: speed
in the form of number
(this is represented as a float value). Its value is 0.2
. The tunable
field allows the roverlib to change this value during runtime of the service.
To access configuration values, you can use the APIs provided by roverlib for your language. We highly recommend using the service.yaml for keeping track of your service parameters.
- Go
- Python
- C
- C++
- Other languages
roverlib-go
exposes the GetFloatSafe()
and GetStringSafe()
methods, that can be used to read a float or string as defined in the service.yaml file, like so:
func run(service roverlib.Service, configuration *roverlib.ServiceConfiguration) error {
tunableSpeed, err := configuration.GetFloatSafe("speed")
if err != nil {
return fmt.Errorf("Failed to get configuration: %v", err)
}
for {
// In your main loop, use GetFloatSafe() every iteration, to make sure you get the latest tuning value
tunableSpeed, err := configuration.GetFloatSafe("speed")
if err != nil {
return fmt.Errorf("Failed to get configuration: %v", err)
}
}
}
roverlib-python
exposes the GetFloatSafe()
and GetStringSafe()
methods, that can be used to read a float or string as defined in the service.yaml file, like so:
def run(service : roverlib.Service, configuration : roverlib.ServiceConfiguration):
tunable_speed = configuration.GetFloatSafe("speed")
if tunable_speed is None:
raise ValueError("Failed to get configuration")
while True:
# In your main loop, use GetFloatSafe() every iteration, to make sure you get the latest tuning value
tunable_speed = configuration.GetFloatSafe("speed")
if tunable_speed is None:
raise ValueError("Failed to get configuration")
roverlib-c
exposes the get_float_value_safe()
and get_string_value_safe()
methods, that can be used to read a float or string as defined in the service.yaml file, like so:
int user_program(Service service, Service_configuration *configuration) {
double *tunable_speed = get_float_value_safe(configuration, "speed");
if (tunable_speed == NULL) {
printf("Failed to get configuration\n");
return 1;
}
while (true) {
// In your main loop, use get_float_value_safe() every iteration, to make sure you get the latest tuning value
double *tunable_speed = get_float_value_safe(configuration, "speed");
if (tunable_speed == NULL) {
printf("Failed to get configuration\n");
return 1;
}
}
}
The C++ template uses roverlib-c
, which exposes the get_float_value_safe()
and get_string_value_safe()
methods, that can be used to read a float or string as defined in the service.yaml file, like so:
int user_program(Service service, Service_configuration *configuration) {
double *tunable_speed = get_float_value_safe(configuration, "speed");
if (tunable_speed == NULL) {
printf("Failed to get configuration\n");
return 1;
}
while (true) {
// In your main loop, use get_float_value_safe() every iteration, to make sure you get the latest tuning value
double *tunable_speed = get_float_value_safe(configuration, "speed");
if (tunable_speed == NULL) {
printf("Failed to get configuration\n");
return 1;
}
}
}
You will need to parse the service information that is injected through the ASE_SERVICE
environment variable manually. This environment variable contains the bootspec which you need to represent in the native format of your language. Then, you need to open a ZMQ socket based on the correct stream properties and you need to receive tuning state messages. It is recommended to look at roverlib-go
's implementation to understand how tuning is done.
Try Tuning
Experiment with reading tunable variables by printing them every iteration. Then, reupload your service and start roverctl-web
in debug mode. Similar to the example pipeline, open the tuning tab and try sending different values to your service. They should be represented in the logs.